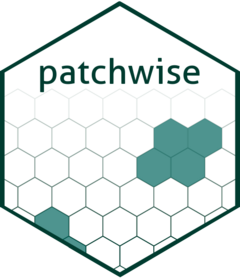
Converts prioritization solution into a more digestible output
convert_solution.Rd
This function converts the prioritization solution that uses patches to a raster or sf object (depending on the format of your spatial_grid) that clearly marks areas suggested for protection (1) and areas not suggested for protection (0)
Arguments
- solution
the solution that results from using
prioritizr::solve()
onprioritizr::problem()
- patch_df
a dataframe generated by
create_patch_df()
that includes constraints for each patch and grid cell combination- spatial_grid
a raster or sf template with the desired resolution and coordinate reference system generated by
spatialgridr::get_grid()
; values in areas of interest are 1, while all other values are NA (only required if feature is a sf object)
Examples
if (FALSE) {
# Start with a little housekeeping to get the data from oceandatr
# Choose area of interest (Bermuda EEZ)
area <- oceandatr::get_area(area_name = "Bermuda", mregions_column = "territory1")
projection <-'+proj=laea +lon_0=-64.8108333 +lat_0=32.3571917 +datum=WGS84 +units=m +no_defs'
# Create a planning grid
planning_raster <- spatialgridr::get_grid(area, projection = projection)
# Grab all relevant data
features_raster <- oceandatr::get_features(spatial_grid = planning_raster)
# Separate seamount data - we want to protect entire patches
seamounts_raster <- features_raster[["seamounts"]]
features_raster <- features_raster[[names(features_raster)[names(features_raster) != "seamounts"]]]
# Create a "cost" to protecting a cell - just a uniform cost for this example
cost_raster <- stats::setNames(planning_raster, "cost")
# Create patches from layer
patches_raster <- create_patches(seamounts_raster)
# Create patch dataframe
patches_raster_df <- create_patch_df(spatial_grid = planning_raster, features = features_raster,
patches = patches_raster, costs = cost_raster)
# Create boundary matrix for prioritizr
boundary_matrix <- create_boundary_matrix(spatial_grid = planning_raster, patches = patches_raster,
patch_df = patches_raster_df)
# Create target features - using just 20% for every feature
features_targets <- features_targets(targets = rep(0.2, (terra::nlyr(features_raster)) + 1),
features = features_raster, pre_patches = seamounts_raster)
# Create constraint targets
constraint_targets <- constraints_targets(feature_targets = features_targets,
patch_df = patches_raster_df)
# Create prioritization problem
problem_raster <- prioritizr::problem(x = patches_raster_df,
features = constraint_targets$feature, cost_column = "cost") %>%
prioritizr::add_min_set_objective() %>%
prioritizr::add_manual_targets(constraint_targets) %>%
prioritizr::add_binary_decisions() %>%
prioritizr::add_boundary_penalties(penalty = 0.000002, data = boundary_matrix) %>%
prioritizr::add_default_solver(gap = 0.1, threads = parallel::detectCores()-1)
# Solve problem
solution <- solve(problem_raster)
# Convert to a more digestible format
suggested_protection <- convert_solution(solution = solution, patch_df = patches_raster_df,
spatial_grid = planning_raster)
}