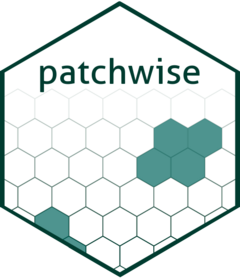
Create patches for a specific feature
create_patches.Rd
This function takes a feature and splits it into multiple "patches" of the feature. This is ideal for features that you want to protect x% of, but want to make sure that entire patches (as opposed to pieces of patches) are protected to meet that target.
Arguments
- feature
a raster or sf object with the feature of interest present (value = 1) or absent (value = NA)
- spatial_grid
a raster or sf template with the desired resolution and coordinate reference system generated by
spatialgridr::get_grid()
; values in areas of interest are 1, while all other values are NA (only required if feature is a sf object)
Value
A raster or sf object with independent layers (raster)/columns (sf) designating the location of each patch
Examples
# Start with a little housekeeping to get the data from oceandatr
# Choose area of interest (Bermuda EEZ)
area <- oceandatr::get_area(area_name = "Bermuda", mregions_column = "territory1")
projection <-'+proj=laea +lon_0=-64.8108333 +lat_0=32.3571917 +datum=WGS84 +units=m +no_defs'
# Create a planning grid
planning_raster <- spatialgridr::get_grid(area, projection = projection)
# Grab all relevant data
features_raster <- oceandatr::get_features(spatial_grid = planning_raster)
#> Getting depth zones...
#> This may take seconds to minutes, depending on grid size
#> Getting seamount data...
#> Spherical geometry (s2) switched off
#> although coordinates are longitude/latitude, st_intersection assumes that they
#> are planar
#> Warning: attribute variables are assumed to be spatially constant throughout all geometries
#> Spherical geometry (s2) switched on
#> Getting knoll data...
#> Warning: attribute variables are assumed to be spatially constant throughout all geometries
#> Getting geomorphology data...
#> Getting coral data...
#> |-- Coral data found for antipatharia coral, cold coral, octocoral
#> Getting environmental regions data... This could take several minutes
# Separate seamount data - we want to protect entire patches
seamounts_raster <- features_raster[["seamounts"]]
features_raster <- features_raster[[names(features_raster)[names(features_raster) != "seamounts"]]]
# Create a "cost" to protecting a cell - just a uniform cost for this example
cost_raster <- stats::setNames(planning_raster, "cost")
# Create patches from layer
patches_raster <- create_patches(seamounts_raster)