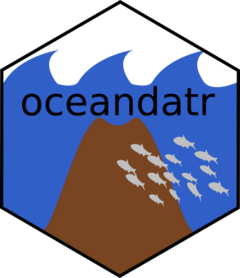
Get an a shapefile for the area of interest
get_area.Rd
This uses the `mrp_get` function from the `mregions2` package to retrieve an area object (e.g. an EEZ) from Marine Regions (https://marineregions.org/gazetteer.php). You can filter using any column available in the `mregions2` database. To see all possible column names and codes, specify `show_options` = TRUE when you run the function.
Usage
get_area(
area_name,
query_type = "eez",
mregions_column = "sovereign1",
show_value_options = FALSE,
show_column_options = FALSE
)
Arguments
- area_name
string; the name of the country or area that you would like to query for; must match a name in `mregions2` for the `mregions_column` selected
- query_type
string; the area type that you would like to query for; some options include "eez", "high_seas", "ecoregions". Run `mregions2::mrp_list` to see the full list of data_product options. (default is "eez")
- mregions_column
string; the name of the column in `mregions2` that you would like to query to find the area corresponding to `area_name` (default is "sovereign1")
- show_value_options
logical; whether to show all of the value options associated with your `mregions_column` selection that are available in `mregions2`; running this will not return a spatial object (default is FALSE)
- show_column_options
logical; whether to show all of the `mregions_column` options that are available in `mregions2`; running this will not return a spatial object (default is FALSE)
Value
if `show_options` = FALSE, returns the sf object of the area of interest; if `show_value_options` or `show_column_options` == TRUE, a named list will be returned. Names in the list correspond to options for `mregions_column` and values correspond to options for `area_name`
Examples
# Show all possible column names
get_area(show_column_options = TRUE)
#> $mregions_column
#> [1] "mrgid" "geoname" "mrgid_ter1" "pol_type" "mrgid_sov1"
#> [6] "territory1" "iso_ter1" "sovereign1" "mrgid_ter2" "mrgid_sov2"
#> [11] "territory2" "iso_ter2" "sovereign2" "mrgid_ter3" "mrgid_sov3"
#> [16] "territory3" "iso_ter3" "sovereign3" "x_1" "y_1"
#> [21] "mrgid_eez" "area_km2" "iso_sov1" "iso_sov2" "iso_sov3"
#> [26] "un_sov1" "un_sov2" "un_sov3" "un_ter1" "un_ter2"
#> [31] "un_ter3"
#>
# Show all possible EEZ areas
get_area(show_value_options = TRUE)
#> $sovereign1
#> [1] "Albania" "Algeria"
#> [3] "Angola" "Antigua and Barbuda"
#> [5] "Argentina" "Australia"
#> [7] "Azerbaijan" "Bahamas"
#> [9] "Bahrain" "Bangladesh"
#> [11] "Barbados" "Belgium"
#> [13] "Belize" "Benin"
#> [15] "Bosnia and Herzegovina" "Brazil"
#> [17] "Brunei" "Bulgaria"
#> [19] "Cambodia" "Cameroon"
#> [21] "Canada" "Cape Verde"
#> [23] "Chile" "China"
#> [25] "Colombia" "Comores"
#> [27] "Costa Rica" "Croatia"
#> [29] "Cuba" "Cyprus"
#> [31] "Democratic Republic of the Congo" "Denmark"
#> [33] "Djibouti" "Dominica"
#> [35] "Dominican Republic" "East Timor"
#> [37] "Ecuador" "Egypt"
#> [39] "El Salvador" "Equatorial Guinea"
#> [41] "Eritrea" "Estonia"
#> [43] "Federal Republic of Somalia" "Fiji"
#> [45] "Finland" "France"
#> [47] "Gabon" "Gambia"
#> [49] "Georgia" "Germany"
#> [51] "Ghana" "Greece"
#> [53] "Grenada" "Guatemala"
#> [55] "Guinea" "Guinea-Bissau"
#> [57] "Guyana" "Haiti"
#> [59] "Honduras" "Iceland"
#> [61] "India" "Indonesia"
#> [63] "Iran" "Iraq"
#> [65] "Ireland" "Israel"
#> [67] "Italy" "Ivory Coast"
#> [69] "Jamaica" "Japan"
#> [71] "Jordan" "Kazakhstan"
#> [73] "Kenya" "Kiribati"
#> [75] "Kuwait" "Latvia"
#> [77] "Lebanon" "Liberia"
#> [79] "Libya" "Lithuania"
#> [81] "Madagascar" "Malaysia"
#> [83] "Maldives" "Malta"
#> [85] "Marshall Islands" "Mauritania"
#> [87] "Mexico" "Micronesia"
#> [89] "Monaco" "Montenegro"
#> [91] "Morocco" "Mozambique"
#> [93] "Myanmar" "Namibia"
#> [95] "Nauru" "Netherlands"
#> [97] "New Zealand" "Nicaragua"
#> [99] "Nigeria" "North Korea"
#> [101] "Norway" "Oman"
#> [103] "Pakistan" "Palau"
#> [105] "Palestine" "Panama"
#> [107] "Papua New Guinea" "Peru"
#> [109] "Philippines" "Poland"
#> [111] "Portugal" "Qatar"
#> [113] "Republic of Mauritius" "Republic of the Congo"
#> [115] "Romania" "Russia"
#> [117] "Saint Kitts and Nevis" "Saint Lucia"
#> [119] "Saint Vincent and the Grenadines" "Samoa"
#> [121] "Sao Tome and Principe" "Saudi Arabia"
#> [123] "Senegal" "Seychelles"
#> [125] "Sierra Leone" "Singapore"
#> [127] "Slovenia" "Solomon Islands"
#> [129] "South Africa" "South Korea"
#> [131] "Spain" "Sri Lanka"
#> [133] "Sudan" "Suriname"
#> [135] "Sweden" "Syria"
#> [137] "São Tomé and Principe" "Taiwan"
#> [139] "Tanzania" "Thailand"
#> [141] "Togo" "Tonga"
#> [143] "Trinidad and Tobago" "Tunisia"
#> [145] "Turkey" "Turkmenistan"
#> [147] "Tuvalu" "Ukraine"
#> [149] "United Arab Emirates" "United Kingdom"
#> [151] "United States" "Uruguay"
#> [153] "Vanuatu" "Venezuela"
#> [155] "Vietnam" "Western Sahara"
#> [157] "Yemen"
#>
# Show all possible options
get_area(show_column_options = TRUE, show_value_options = TRUE)
#> Please be patient, this may take some time.
#> $mrgid
#> [1] 3293 5668 5669 5670 5672 5673 5674 5675 5676 5677 5678 5679
#> [13] 5680 5681 5682 5683 5684 5685 5686 5687 5688 5689 5690 5691
#> [25] 5692 5693 5694 5695 5696 5697 8308 8309 8310 8311 8312 8313
#> [37] 8314 8315 8316 8317 8318 8319 8321 8322 8323 8324 8325 8326
#> [49] 8327 8328 8331 8332 8333 8334 8337 8338 8339 8340 8341 8343
#> [61] 8345 8346 8347 8348 8349 8350 8351 8352 8353 8354 8355 8356
#> [73] 8357 8358 8359 8360 8361 8362 8363 8364 8365 8366 8367 8368
#> [85] 8369 8370 8371 8372 8373 8374 8375 8376 8378 8379 8380 8381
#> [97] 8382 8383 8384 8385 8386 8387 8388 8389 8390 8391 8392 8393
#> [109] 8394 8395 8396 8397 8398 8400 8401 8402 8403 8404 8405 8406
#> [121] 8407 8408 8409 8411 8412 8413 8414 8415 8416 8417 8418 8419
#> [133] 8420 8421 8423 8424 8425 8426 8427 8428 8429 8430 8431 8432
#> [145] 8433 8435 8437 8438 8439 8440 8441 8442 8443 8444 8445 8446
#> [157] 8447 8448 8449 8450 8451 8452 8453 8454 8455 8456 8457 8459
#> [169] 8460 8461 8462 8463 8464 8465 8466 8467 8468 8469 8470 8471
#> [181] 8472 8473 8474 8475 8476 8477 8478 8479 8480 8481 8482 8483
#> [193] 8484 8485 8486 8487 8488 8490 8491 8492 8493 8494 8495 8758
#> [205] 21787 21788 21789 21790 21791 21792 21796 21797 21798 21803 22491 22756
#> [217] 26517 26518 26519 26520 26521 26522 26523 26524 26526 26582 33177 33178
#> [229] 33179 33180 33181 33185 48943 48944 48945 48946 48947 48948 48950 48951
#> [241] 48952 48953 48954 48955 48956 48957 48961 48962 48964 48965 48966 48967
#> [253] 48968 48969 48970 48971 48972 48973 48974 48975 48976 48977 48978 48980
#> [265] 48982 48984 48985 48997 48998 48999 49000 49001 49002 49003 50167 50170
#> [277] 62589 62596 62598 64430 64431 64440 64446 64459 64460
#>
#> $geoname
#> [1] "Albanian Exclusive Economic Zone"
#> [2] "Algerian Exclusive Economic Zone"
#> [3] "Angolan Exclusive Economic Zone"
#> [4] "Antiguan and Barbudan Exclusive Economic Zone"
#> [5] "Argentinian Exclusive Economic Zone"
#> [6] "Australian Exclusive Economic Zone"
#> [7] "Australian Exclusive Economic Zone (Christmas Island)"
#> [8] "Australian Exclusive Economic Zone (Cocos Islands)"
#> [9] "Australian Exclusive Economic Zone (Heard and McDonald Islands)"
#> [10] "Australian Exclusive Economic Zone (Macquarie Island)"
#> [11] "Australian Exclusive Economic Zone (Norfolk Island)"
#> [12] "Azerbaijani Exclusive Economic Zone"
#> [13] "Bahamian Exclusive Economic Zone"
#> [14] "Bahraini Exclusive Economic Zone"
#> [15] "Bangladeshi Exclusive Economic Zone"
#> [16] "Barbadian Exclusive Economic Zone"
#> [17] "Belgian Exclusive Economic Zone"
#> [18] "Belizean Exclusive Economic Zone"
#> [19] "Beninese Exclusive Economic Zone"
#> [20] "Bissau-Guinean Exclusive Economic Zone"
#> [21] "Bosnian and Herzegovinian Exclusive Economic Zone"
#> [22] "Brazilian Exclusive Economic Zone"
#> [23] "Brazilian Exclusive Economic Zone (Trindade)"
#> [24] "British Exclusive Economic Zone"
#> [25] "British Exclusive Economic Zone (Anguilla)"
#> [26] "British Exclusive Economic Zone (Ascension)"
#> [27] "British Exclusive Economic Zone (Bermuda)"
#> [28] "British Exclusive Economic Zone (British Virgin Islands)"
#> [29] "British Exclusive Economic Zone (Cayman Islands)"
#> [30] "British Exclusive Economic Zone (Guernsey)"
#> [31] "British Exclusive Economic Zone (Isle of Man)"
#> [32] "British Exclusive Economic Zone (Jersey)"
#> [33] "British Exclusive Economic Zone (Montserrat)"
#> [34] "British Exclusive Economic Zone (Pitcairn)"
#> [35] "British Exclusive Economic Zone (Saint Helena)"
#> [36] "British Exclusive Economic Zone (Tristan da Cunha)"
#> [37] "British Exclusive Economic Zone (Turks and Caicos Islands)"
#> [38] "Bruneian Exclusive Economic Zone"
#> [39] "Bulgarian Exclusive Economic Zone"
#> [40] "Cambodian Exclusive Economic Zone"
#> [41] "Cameroonian Exclusive Economic Zone"
#> [42] "Canadian Exclusive Economic Zone"
#> [43] "Cape Verdean Exclusive Economic Zone"
#> [44] "Chilean Exclusive Economic Zone"
#> [45] "Chilean Exclusive Economic Zone (Easter Island)"
#> [46] "Chilean Exclusive Economic Zone (Islas San Félix and San Ambrosio)"
#> [47] "Chinese Exclusive Economic Zone"
#> [48] "Colombian Exclusive Economic Zone"
#> [49] "Colombian Exclusive Economic Zone (Bajo Nuevo Bank)"
#> [50] "Colombian Exclusive Economic Zone (Quitasueño Bank)"
#> [51] "Colombian Exclusive Economic Zone (Serrana Bank)"
#> [52] "Colombian Exclusive Economic Zone (Serranilla Bank)"
#> [53] "Comoran Exclusive Economic Zone"
#> [54] "Congolese (Democratic Republic of) Exclusive Economic Zone"
#> [55] "Congolese (Republic of) Exclusive Economic Zone"
#> [56] "Costa Rican Exclusive Economic Zone"
#> [57] "Croatian Exclusive Economic Zone"
#> [58] "Cuban Exclusive Economic Zone"
#> [59] "Cypriote Exclusive Economic Zone"
#> [60] "Danish Exclusive Economic Zone"
#> [61] "Danish Exclusive Economic Zone (Faeroe)"
#> [62] "Danish Exclusive Economic Zone (Greenland)"
#> [63] "Djiboutian Exclusive Economic Zone"
#> [64] "Dominican (Republic) Exclusive Economic Zone"
#> [65] "Dominican Exclusive Economic Zone"
#> [66] "Dutch Exclusive Economic Zone"
#> [67] "Dutch Exclusive Economic Zone (Aruba)"
#> [68] "Dutch Exclusive Economic Zone (Bonaire)"
#> [69] "Dutch Exclusive Economic Zone (Curaçao)"
#> [70] "Dutch Exclusive Economic Zone (Saba)"
#> [71] "Dutch Exclusive Economic Zone (Sint-Eustatius)"
#> [72] "Dutch Exclusive Economic Zone (Sint-Maarten)"
#> [73] "Ecuadorian Exclusive Economic Zone"
#> [74] "Ecuadorian Exclusive Economic Zone (Galapagos)"
#> [75] "Egyptian Exclusive Economic Zone"
#> [76] "Emirati Exclusive Economic Zone"
#> [77] "Equatorial Guinean Exclusive Economic Zone"
#> [78] "Eritrean Exclusive Economic Zone"
#> [79] "Estonian Exclusive Economic Zone"
#> [80] "Fijian Exclusive Economic Zone"
#> [81] "Finnish Exclusive Economic Zone"
#> [82] "French Exclusive Economic Zone"
#> [83] "French Exclusive Economic Zone (Amsterdam and Saint Paul Islands)"
#> [84] "French Exclusive Economic Zone (Bassas da India)"
#> [85] "French Exclusive Economic Zone (Clipperton Island)"
#> [86] "French Exclusive Economic Zone (Collectivity of Saint Martin)"
#> [87] "French Exclusive Economic Zone (Crozet Islands)"
#> [88] "French Exclusive Economic Zone (Europa Island)"
#> [89] "French Exclusive Economic Zone (French Guiana)"
#> [90] "French Exclusive Economic Zone (French Polynesia)"
#> [91] "French Exclusive Economic Zone (Guadeloupe)"
#> [92] "French Exclusive Economic Zone (Juan de Nova Island)"
#> [93] "French Exclusive Economic Zone (Kerguélen)"
#> [94] "French Exclusive Economic Zone (Martinique)"
#> [95] "French Exclusive Economic Zone (New Caledonia)"
#> [96] "French Exclusive Economic Zone (Réunion)"
#> [97] "French Exclusive Economic Zone (Saint-Barthélemy)"
#> [98] "French Exclusive Economic Zone (Saint-Pierre and Miquelon)"
#> [99] "French Exclusive Economic Zone (Wallis and Futuna)"
#> [100] "Gabonese Exclusive Economic Zone"
#> [101] "Gambian Exclusive Economic Zone"
#> [102] "Georgian Exclusive Economic Zone"
#> [103] "German Exclusive Economic Zone"
#> [104] "Ghanaian Exclusive Economic Zone"
#> [105] "Greek Exclusive Economic Zone"
#> [106] "Grenadian Exclusive Economic Zone"
#> [107] "Guatemalan Exclusive Economic Zone"
#> [108] "Guinean Exclusive Economic Zone"
#> [109] "Guyanese Exclusive Economic Zone"
#> [110] "Haitian Exclusive Economic Zone"
#> [111] "Honduran Exclusive Economic Zone"
#> [112] "Icelandic Exclusive Economic Zone"
#> [113] "Indian Exclusive Economic Zone"
#> [114] "Indian Exclusive Economic Zone (Andaman and Nicobar Islands)"
#> [115] "Indonesian Exclusive Economic Zone"
#> [116] "Iranian Exclusive Economic Zone"
#> [117] "Iraqi Exclusive Economic Zone"
#> [118] "Irish Exclusive Economic Zone"
#> [119] "Israeli Exclusive Economic Zone"
#> [120] "Italian Exclusive Economic Zone"
#> [121] "Ivorian Exclusive Economic Zone"
#> [122] "Jamaican Exclusive Economic Zone"
#> [123] "Japanese Exclusive Economic Zone"
#> [124] "Joint regime area Torres Strait Treaty: Papua New Guinea / Australia"
#> [125] "Joint regime area: Barbados / Guyana"
#> [126] "Joint regime area: Costa Rica / Ecuador (Galapagos)"
#> [127] "Joint regime area: Croatia / Slovenia"
#> [128] "Joint regime area: Dominican Republic / Colombia"
#> [129] "Joint regime area: Ecuador / Colombia"
#> [130] "Joint regime area: France / Italy"
#> [131] "Joint regime area: Honduras / United Kingdom (Cayman Islands)"
#> [132] "Joint regime area: Iceland / Denmark (Faeroe Islands)"
#> [133] "Joint regime area: Iceland / Norway (Jan Mayen)"
#> [134] "Joint regime area: Jamaica / Colombia"
#> [135] "Joint regime area: Norway / Russia"
#> [136] "Joint regime area: Peru / Ecuador"
#> [137] "Joint regime area: Senegal / Guinea-Bissau"
#> [138] "Joint regime area: South Korea / Japan"
#> [139] "Joint regime area: Spain / France"
#> [140] "Joint regime area: Sweden / Norway"
#> [141] "Joint regime area: São Tomé and Principe / Nigeria"
#> [142] "Joint regime area: United Kingdom / Denmark (Faeroe Islands)"
#> [143] "Joint regime area: United States / Russia"
#> [144] "Joint regime area: Uruguay / Argentina"
#> [145] "Jordanian Exclusive Economic Zone"
#> [146] "Kazakh Exclusive Economic Zone"
#> [147] "Kenyan Exclusive Economic Zone"
#> [148] "Kiribati Exclusive Economic Zone (Gilbert Islands)"
#> [149] "Kiribati Exclusive Economic Zone (Line Group)"
#> [150] "Kiribati Exclusive Economic Zone (Phoenix Group)"
#> [151] "Kittitian and Nevisian Exclusive Economic Zone"
#> [152] "Kuwaiti Exclusive Economic Zone"
#> [153] "Latvian Exclusive Economic Zone"
#> [154] "Lebanese Exclusive Economic Zone"
#> [155] "Liberian Exclusive Economic Zone"
#> [156] "Libyan Exclusive Economic Zone"
#> [157] "Lithuanian Exclusive Economic Zone"
#> [158] "Madagascan Exclusive Economic Zone"
#> [159] "Malaysian Exclusive Economic Zone"
#> [160] "Maldivian Exclusive Economic Zone"
#> [161] "Maltese Exclusive Economic Zone"
#> [162] "Marshallese Exclusive Economic Zone"
#> [163] "Mauritanian Exclusive Economic Zone"
#> [164] "Mauritian Exclusive Economic Zone"
#> [165] "Mauritian Exclusive Economic Zone (Chagos Archipelago)"
#> [166] "Mexican Exclusive Economic Zone"
#> [167] "Micronesian Exclusive Economic Zone"
#> [168] "Montenegrin Exclusive Economic Zone"
#> [169] "Monégasque Exclusive Economic Zone"
#> [170] "Moroccan Exclusive Economic Zone"
#> [171] "Mozambican Exclusive Economic Zone"
#> [172] "Myanma Exclusive Economic Zone"
#> [173] "Namibian Exclusive Economic Zone"
#> [174] "Nauruan Exclusive Economic Zone"
#> [175] "New Zealand Exclusive Economic Zone"
#> [176] "New Zealand Exclusive Economic Zone (Cook Islands)"
#> [177] "New Zealand Exclusive Economic Zone (Niue)"
#> [178] "New Zealand Exclusive Economic Zone (Tokelau)"
#> [179] "Nicaraguan Exclusive Economic Zone"
#> [180] "Nigerian Exclusive Economic Zone"
#> [181] "North Korean Exclusive Economic Zone"
#> [182] "Norwegian Exclusive Economic Zone"
#> [183] "Norwegian Exclusive Economic Zone (Jan Mayen)"
#> [184] "Norwegian Exclusive Economic Zone (Svalbard)"
#> [185] "Omani Exclusive Economic Zone"
#> [186] "Overlapping claim Abu musa, Greater and Lesser Tunb: United Arab Emirates / Iran"
#> [187] "Overlapping claim Alhucemas Islands: Spain / Morocco"
#> [188] "Overlapping claim Ceuta: Spain / Morocco"
#> [189] "Overlapping claim Chafarinas Islands: Spain / Morocco"
#> [190] "Overlapping claim Doumeira Islands: Eritrea / Djibouti"
#> [191] "Overlapping claim Falkland / Malvinas Islands: United Kingdom / Argentina"
#> [192] "Overlapping claim Gibraltar: United Kingdom / Spain"
#> [193] "Overlapping claim Glorioso Islands: France / Madagascar"
#> [194] "Overlapping claim Halaib Triangle: Sudan / Egypt"
#> [195] "Overlapping claim Ile Tromelin: France / Madagascar / Republic of Mauritius"
#> [196] "Overlapping claim Kuril Islands: Japan / Russia"
#> [197] "Overlapping claim Liancourt Rocks: Japan / South Korea"
#> [198] "Overlapping claim Matthew and Hunter Islands: France / Vanuatu"
#> [199] "Overlapping claim Mayotte: France / Comores"
#> [200] "Overlapping claim Melilla: Spain / Morocco"
#> [201] "Overlapping claim Navassa Island: United States / Haiti / Jamaica"
#> [202] "Overlapping claim Perejil Island: Spain / Morocco"
#> [203] "Overlapping claim Peñón de Vélez de la Gomera: Spain / Morocco"
#> [204] "Overlapping claim Senkaku Islands: Taiwan / Japan / China"
#> [205] "Overlapping claim South Georgia and the South Sandwich Islands: United Kingdom / Argentina"
#> [206] "Overlapping claim Taiwan: Taiwan / China"
#> [207] "Overlapping claim Ukraine: Ukraine / Russia"
#> [208] "Overlapping claim Wake Island / Enenkio: United States / Marshall Islands"
#> [209] "Overlapping claim Western Sahara: Western Sahara / Morocco"
#> [210] "Overlapping claim: Belize / Honduras"
#> [211] "Overlapping claim: Canada / United States"
#> [212] "Overlapping claim: Colombia / Dominican Republic / Venezuela"
#> [213] "Overlapping claim: Egypt / Libya"
#> [214] "Overlapping claim: Guyana / Trinidad and Tobago / Venezuela"
#> [215] "Overlapping claim: Iraq / Iran"
#> [216] "Overlapping claim: Malaysia / Singapore"
#> [217] "Overlapping claim: Qatar / Saudi Arabia / United Arab Emirates"
#> [218] "Overlapping claim: South China Sea"
#> [219] "Overlapping claim: United States (Puerto Rico) / Dominican Republic"
#> [220] "Overlapping claim: Venezuela / Netherlands (Aruba) / Dominican Republic"
#> [221] "Pakistani Exclusive Economic Zone"
#> [222] "Palauan Exclusive Economic Zone"
#> [223] "Palestinian Exclusive Economic Zone"
#> [224] "Panamanian Exclusive Economic Zone"
#> [225] "Papua New Guinean Exclusive Economic Zone"
#> [226] "Peruvian Exclusive Economic Zone"
#> [227] "Philippine Exclusive Economic Zone"
#> [228] "Polish Exclusive Economic Zone"
#> [229] "Portuguese Exclusive Economic Zone"
#> [230] "Portuguese Exclusive Economic Zone (Azores)"
#> [231] "Portuguese Exclusive Economic Zone (Madeira)"
#> [232] "Qatari Exclusive Economic Zone"
#> [233] "Romanian Exclusive Economic Zone"
#> [234] "Russian Exclusive Economic Zone"
#> [235] "Saint Lucian Exclusive Economic Zone"
#> [236] "Saint Vincentian Exclusive Economic Zone"
#> [237] "Salvadoran Exclusive Economic Zone"
#> [238] "Samoan Exclusive Economic Zone"
#> [239] "Saudi Arabian Exclusive Economic Zone"
#> [240] "Senegalese Exclusive Economic Zone"
#> [241] "Seychellois Exclusive Economic Zone"
#> [242] "Sierra Leonean Exclusive Economic Zone"
#> [243] "Singaporean Exclusive Economic Zone"
#> [244] "Slovenian Exclusive Economic Zone"
#> [245] "Solomon Islands Exclusive Economic Zone"
#> [246] "Somali Exclusive Economic Zone"
#> [247] "South African Exclusive Economic Zone"
#> [248] "South African Exclusive Economic Zone (Prince Edward Islands)"
#> [249] "South Korean Exclusive Economic Zone"
#> [250] "Spanish Exclusive Economic Zone"
#> [251] "Spanish Exclusive Economic Zone (Canary Islands)"
#> [252] "Sri Lankan Exclusive Economic Zone"
#> [253] "Sudanese Exclusive Economic Zone"
#> [254] "Surinamese Exclusive Economic Zone"
#> [255] "Swedish Exclusive Economic Zone"
#> [256] "Syrian Exclusive Economic Zone"
#> [257] "São Toméan Exclusive Economic Zone"
#> [258] "Tanzanian Exclusive Economic Zone"
#> [259] "Thailand Exclusive Economic Zone"
#> [260] "Timorese Exclusive Economic Zone"
#> [261] "Timorese Exclusive Economic Zone (Oecusse)"
#> [262] "Togolese Exclusive Economic Zone"
#> [263] "Tongan Exclusive Economic Zone"
#> [264] "Trinidadian and Tobagonian Exclusive Economic Zone"
#> [265] "Tunisian Exclusive Economic Zone"
#> [266] "Turkish Exclusive Economic Zone"
#> [267] "Turkmen Exclusive Economic Zone"
#> [268] "Tuvaluan Exclusive Economic Zone"
#> [269] "United States Exclusive Economic Zone"
#> [270] "United States Exclusive Economic Zone (Alaska)"
#> [271] "United States Exclusive Economic Zone (American Samoa)"
#> [272] "United States Exclusive Economic Zone (Guam)"
#> [273] "United States Exclusive Economic Zone (Hawaii)"
#> [274] "United States Exclusive Economic Zone (Howland and Baker Islands)"
#> [275] "United States Exclusive Economic Zone (Jarvis Islands)"
#> [276] "United States Exclusive Economic Zone (Johnston Atoll)"
#> [277] "United States Exclusive Economic Zone (Northern Mariana Islands)"
#> [278] "United States Exclusive Economic Zone (Palmyra Atoll)"
#> [279] "United States Exclusive Economic Zone (Puerto Rico)"
#> [280] "United States Exclusive Economic Zone (United States Virgin Islands)"
#> [281] "Uruguayan Exclusive Economic Zone"
#> [282] "Vanuatuan Exclusive Economic Zone"
#> [283] "Venezuelan Exclusive Economic Zone"
#> [284] "Vietnamese Exclusive Economic Zone"
#> [285] "Yemeni Exclusive Economic Zone"
#>
#> $mrgid_ter1
#> [1] 14 15 17 2097 2098 2099 2100 2101 2102 2103 2104 2105
#> [13] 2106 2108 2110 2111 2112 2113 2114 2115 2118 2119 2120 2121
#> [25] 2122 2123 2124 2125 2126 2127 2128 2129 2131 2132 2133 2134
#> [37] 2135 2136 2139 2142 2143 2144 2145 2147 2149 2150 2152 2153
#> [49] 2154 2156 2157 2159 2160 2161 2162 2163 2165 2166 2168 2169
#> [61] 2170 2171 2174 2175 2176 2177 2180 2182 2183 2185 2186 2187
#> [73] 2188 2190 2191 2192 2194 2195 2196 2198 2199 2200 2201 2202
#> [85] 2204 2205 2206 2207 2208 2210 2211 2212 2213 2214 2215 2216
#> [97] 2217 2218 2219 2220 2221 2222 2224 2225 2226 2227 2229 2230
#> [109] 2236 2237 2238 2240 2241 2242 2243 2244 2245 2249 2250 2251
#> [121] 2252 2253 2255 2257 2259 2260 2261 2454 3297 3344 3384 3743
#> [133] 3868 4377 4378 4530 4956 5334 5343 5363 5660 5765 5770 5772
#> [145] 7963 8468 8589 8590 8591 8592 8593 8594 8595 8596 8597 8598
#> [157] 8599 8600 8601 8603 8605 8606 8607 8609 8610 8612 8613 8614
#> [169] 8616 8617 8619 8620 8621 8622 8623 8624 8627 8630 8631 8632
#> [181] 8633 8635 8636 8637 8638 8639 8640 8641 8642 8643 8644 8645
#> [193] 8646 8647 8648 8649 8650 8651 8652 8653 8654 8655 8656 8657
#> [205] 8658 8668 8669 8670 8671 8672 8673 8674 8675 8678 8679 8680
#> [217] 8681 8682 8683 8684 8685 8686 8688 8757 9011 14864 15074 15120
#> [229] 15525 17597 17831 19095 19099 19143 20312 21802 22598 25135 26234 26525
#> [241] 31136 36288 48684 48934 48935 48936 48979 48983 48994 48995 48996 49593
#> [253] 64457 64458
#>
#> $pol_type
#> [1] "200NM" "Joint regime" "Overlapping claim"
#>
#> $mrgid_sov1
#> [1] 14 15 17 2097 2098 2099 2100 2101 2102 2103 2104 2105
#> [13] 2106 2108 2110 2111 2112 2113 2114 2115 2116 2118 2119 2120
#> [25] 2121 2122 2123 2124 2125 2126 2127 2128 2129 2131 2132 2133
#> [37] 2134 2135 2136 2139 2142 2143 2144 2145 2147 2149 2150 2152
#> [49] 2153 2154 2156 2157 2159 2160 2161 2162 2163 2165 2166 2168
#> [61] 2169 2170 2171 2174 2175 2176 2177 2180 2182 2183 2185 2186
#> [73] 2187 2188 2190 2191 2192 2194 2195 2196 2198 2199 2200 2201
#> [85] 2202 2204 2205 2206 2207 2208 2210 2212 2213 2214 2215 2216
#> [97] 2217 2218 2219 2220 2221 2222 2224 2225 2226 2227 2229 2230
#> [109] 2236 2237 2238 2240 2241 2242 2243 2244 2245 2249 2250 2251
#> [121] 2252 2253 2255 2257 3384 3868 7963 8468 8593 8594 8595 8596
#> [133] 8600 8601 8603 8614 8617 8632 8633 8637 8639 8640 8644 8645
#> [145] 8647 8648 8649 8650 8651 8671 8674 8681 8682 8685 8757 15525
#> [157] 17831
#>
#> $territory1
#> [1] "Abu musa, Greater and Lesser Tunb"
#> [2] "Alaska"
#> [3] "Albania"
#> [4] "Algeria"
#> [5] "Alhucemas Islands"
#> [6] "American Samoa"
#> [7] "Amsterdam and Saint Paul Islands"
#> [8] "Andaman and Nicobar"
#> [9] "Angola"
#> [10] "Anguilla"
#> [11] "Antigua and Barbuda"
#> [12] "Argentina"
#> [13] "Aruba"
#> [14] "Ascension"
#> [15] "Australia"
#> [16] "Azerbaijan"
#> [17] "Azores"
#> [18] "Bahamas"
#> [19] "Bahrain"
#> [20] "Bajo Nuevo Bank"
#> [21] "Bangladesh"
#> [22] "Barbados"
#> [23] "Bassas da India"
#> [24] "Belgium"
#> [25] "Belize"
#> [26] "Benin"
#> [27] "Bermuda"
#> [28] "Bonaire"
#> [29] "Bosnia and Herzegovina"
#> [30] "Brazil"
#> [31] "British Virgin Islands"
#> [32] "Brunei"
#> [33] "Bulgaria"
#> [34] "Cambodia"
#> [35] "Cameroon"
#> [36] "Canada"
#> [37] "Canary Islands"
#> [38] "Cape Verde"
#> [39] "Cayman Islands"
#> [40] "Ceuta"
#> [41] "Chafarinas Islands"
#> [42] "Chagos Archipelago"
#> [43] "Chile"
#> [44] "China"
#> [45] "Christmas Island"
#> [46] "Clipperton Island"
#> [47] "Cocos Islands"
#> [48] "Collectivity of Saint Martin"
#> [49] "Colombia"
#> [50] "Comores"
#> [51] "Cook Islands"
#> [52] "Costa Rica"
#> [53] "Croatia"
#> [54] "Crozet Islands"
#> [55] "Cuba"
#> [56] "Curaçao"
#> [57] "Cyprus"
#> [58] "Democratic Republic of the Congo"
#> [59] "Denmark"
#> [60] "Djibouti"
#> [61] "Dominica"
#> [62] "Dominican Republic"
#> [63] "Doumeira Islands"
#> [64] "East Timor"
#> [65] "Easter Island"
#> [66] "Ecuador"
#> [67] "Egypt"
#> [68] "El Salvador"
#> [69] "Equatorial Guinea"
#> [70] "Eritrea"
#> [71] "Estonia"
#> [72] "Europa Island"
#> [73] "Faeroe"
#> [74] "Falkland / Malvinas Islands"
#> [75] "Federal Republic of Somalia"
#> [76] "Fiji"
#> [77] "Finland"
#> [78] "France"
#> [79] "French Guiana"
#> [80] "French Polynesia"
#> [81] "Gabon"
#> [82] "Galapagos"
#> [83] "Gambia"
#> [84] "Georgia"
#> [85] "Germany"
#> [86] "Ghana"
#> [87] "Gibraltar"
#> [88] "Gilbert Islands"
#> [89] "Glorioso Islands"
#> [90] "Greece"
#> [91] "Greenland"
#> [92] "Grenada"
#> [93] "Guadeloupe"
#> [94] "Guam"
#> [95] "Guatemala"
#> [96] "Guernsey"
#> [97] "Guinea"
#> [98] "Guinea-Bissau"
#> [99] "Guyana"
#> [100] "Haiti"
#> [101] "Halaib Triangle"
#> [102] "Hawaii"
#> [103] "Heard and McDonald Islands"
#> [104] "Honduras"
#> [105] "Howland and Baker Islands"
#> [106] "Iceland"
#> [107] "Ile Tromelin"
#> [108] "India"
#> [109] "Indonesia"
#> [110] "Iran"
#> [111] "Iraq"
#> [112] "Ireland"
#> [113] "Islas San Félix and San Ambrosio"
#> [114] "Isle of Man"
#> [115] "Israel"
#> [116] "Italy"
#> [117] "Ivory Coast"
#> [118] "Jamaica"
#> [119] "Jan Mayen"
#> [120] "Japan"
#> [121] "Jarvis Island"
#> [122] "Jersey"
#> [123] "Johnston Atoll"
#> [124] "Jordan"
#> [125] "Juan de Nova Island"
#> [126] "Kazakhstan"
#> [127] "Kenya"
#> [128] "Kerguélen"
#> [129] "Kuril Islands"
#> [130] "Kuwait"
#> [131] "Latvia"
#> [132] "Lebanon"
#> [133] "Liancourt Rocks"
#> [134] "Liberia"
#> [135] "Libya"
#> [136] "Line Group"
#> [137] "Lithuania"
#> [138] "Macquarie Island"
#> [139] "Madagascar"
#> [140] "Madeira"
#> [141] "Malaysia"
#> [142] "Maldives"
#> [143] "Malta"
#> [144] "Marshall Islands"
#> [145] "Martinique"
#> [146] "Matthew and Hunter Islands"
#> [147] "Mauritania"
#> [148] "Mayotte"
#> [149] "Melilla"
#> [150] "Mexico"
#> [151] "Micronesia"
#> [152] "Monaco"
#> [153] "Montenegro"
#> [154] "Montserrat"
#> [155] "Morocco"
#> [156] "Mozambique"
#> [157] "Myanmar"
#> [158] "Namibia"
#> [159] "Nauru"
#> [160] "Navassa Island"
#> [161] "Netherlands"
#> [162] "New Caledonia"
#> [163] "New Zealand"
#> [164] "Nicaragua"
#> [165] "Nigeria"
#> [166] "Niue"
#> [167] "Norfolk Island"
#> [168] "North Korea"
#> [169] "Northern Mariana Islands"
#> [170] "Norway"
#> [171] "Oecusse"
#> [172] "Oman"
#> [173] "Pakistan"
#> [174] "Palau"
#> [175] "Palestine"
#> [176] "Palmyra Atoll"
#> [177] "Panama"
#> [178] "Papua New Guinea"
#> [179] "Perejil Island"
#> [180] "Peru"
#> [181] "Peñón de Vélez de la Gomera"
#> [182] "Philippines"
#> [183] "Phoenix Group"
#> [184] "Pitcairn"
#> [185] "Poland"
#> [186] "Portugal"
#> [187] "Prince Edward Islands"
#> [188] "Puerto Rico"
#> [189] "Qatar"
#> [190] "Quitasueño Bank"
#> [191] "Republic of Mauritius"
#> [192] "Republic of the Congo"
#> [193] "Romania"
#> [194] "Russia"
#> [195] "Réunion"
#> [196] "Saba"
#> [197] "Saint Helena"
#> [198] "Saint Kitts and Nevis"
#> [199] "Saint Lucia"
#> [200] "Saint Vincent and the Grenadines"
#> [201] "Saint-Barthélemy"
#> [202] "Saint-Pierre and Miquelon"
#> [203] "Samoa"
#> [204] "Sao Tome and Principe"
#> [205] "Saudi Arabia"
#> [206] "Senegal"
#> [207] "Senkaku Islands"
#> [208] "Serrana Bank"
#> [209] "Serranilla Bank"
#> [210] "Seychelles"
#> [211] "Sierra Leone"
#> [212] "Singapore"
#> [213] "Sint-Eustatius"
#> [214] "Sint-Maarten"
#> [215] "Slovenia"
#> [216] "Solomon Islands"
#> [217] "South Africa"
#> [218] "South Georgia and the South Sandwich Islands"
#> [219] "South Korea"
#> [220] "Spain"
#> [221] "Sri Lanka"
#> [222] "Sudan"
#> [223] "Suriname"
#> [224] "Svalbard"
#> [225] "Sweden"
#> [226] "Syria"
#> [227] "São Tomé and Principe"
#> [228] "Taiwan"
#> [229] "Tanzania"
#> [230] "Thailand"
#> [231] "Togo"
#> [232] "Tokelau"
#> [233] "Tonga"
#> [234] "Trindade"
#> [235] "Trinidad and Tobago"
#> [236] "Tristan da Cunha"
#> [237] "Tunisia"
#> [238] "Turkey"
#> [239] "Turkmenistan"
#> [240] "Turks and Caicos Islands"
#> [241] "Tuvalu"
#> [242] "Ukraine"
#> [243] "United Arab Emirates"
#> [244] "United Kingdom"
#> [245] "United States"
#> [246] "United States Virgin Islands"
#> [247] "Uruguay"
#> [248] "Vanuatu"
#> [249] "Venezuela"
#> [250] "Vietnam"
#> [251] "Wake Island / Enenkio"
#> [252] "Wallis and Futuna"
#> [253] "Western Sahara"
#> [254] "Yemen"
#>
#> $iso_ter1
#> [1] "ABW" "AGO" "AIA" "ALB" "ARE" "ARG" "ASM" "ATF" "ATG" "AUS" "AZE" "BEL"
#> [13] "BEN" "BES" "BGD" "BGR" "BHR" "BHS" "BIH" "BLM" "BLZ" "BMU" "BRA" "BRB"
#> [25] "BRN" "CAN" "CCK" "CHL" "CHN" "CIV" "CMR" "COD" "COG" "COK" "COL" "COM"
#> [37] "CPV" "CRI" "CUB" "CUW" "CXR" "CYM" "CYP" "DEU" "DJI" "DMA" "DNK" "DOM"
#> [49] "DZA" "ECU" "EGY" "ERI" "ESH" "ESP" "EST" "FIN" "FJI" "FLK" "FRA" "FRO"
#> [61] "FSM" "GAB" "GBR" "GEO" "GGY" "GHA" "GIB" "GIN" "GLP" "GMB" "GNB" "GNQ"
#> [73] "GRC" "GRD" "GRL" "GTM" "GUF" "GUM" "GUY" "HMD" "HND" "HRV" "HTI" "IDN"
#> [85] "IMN" "IND" "IRL" "IRN" "IRQ" "ISL" "ISR" "ITA" "JAM" "JEY" "JOR" "JPN"
#> [97] "KAZ" "KEN" "KHM" "KIR" "KNA" "KOR" "KWT" "LBN" "LBR" "LBY" "LCA" "LKA"
#> [109] "LTU" "LVA" "MAF" "MAR" "MCO" "MDG" "MDV" "MEX" "MHL" "MLT" "MMR" "MNE"
#> [121] "MNP" "MOZ" "MRT" "MSR" "MTQ" "MUS" "MYS" "MYT" "NAM" "NCL" "NFK" "NGA"
#> [133] "NIC" "NIU" "NLD" "NOR" "NRU" "NZL" "OMN" "PAK" "PAN" "PCN" "PER" "PHL"
#> [145] "PLW" "PNG" "POL" "PRI" "PRK" "PRT" "PSE" "PYF" "QAT" "REU" "ROU" "RUS"
#> [157] "SAU" "SDN" "SEN" "SGP" "SGS" "SHN" "SJM" "SLB" "SLE" "SLV" "SOM" "SPM"
#> [169] "STP" "SUR" "SVN" "SWE" "SXM" "SYC" "SYR" "TCA" "TGO" "THA" "TKL" "TKM"
#> [181] "TLS" "TON" "TTO" "TUN" "TUR" "TUV" "TWN" "TZA" "UKR" "UMI" "URY" "USA"
#> [193] "VCT" "VEN" "VGB" "VIR" "VNM" "VUT" "WLF" "WSM" "YEM" "ZAF"
#>
#> $sovereign1
#> [1] "Albania" "Algeria"
#> [3] "Angola" "Antigua and Barbuda"
#> [5] "Argentina" "Australia"
#> [7] "Azerbaijan" "Bahamas"
#> [9] "Bahrain" "Bangladesh"
#> [11] "Barbados" "Belgium"
#> [13] "Belize" "Benin"
#> [15] "Bosnia and Herzegovina" "Brazil"
#> [17] "Brunei" "Bulgaria"
#> [19] "Cambodia" "Cameroon"
#> [21] "Canada" "Cape Verde"
#> [23] "Chile" "China"
#> [25] "Colombia" "Comores"
#> [27] "Costa Rica" "Croatia"
#> [29] "Cuba" "Cyprus"
#> [31] "Democratic Republic of the Congo" "Denmark"
#> [33] "Djibouti" "Dominica"
#> [35] "Dominican Republic" "East Timor"
#> [37] "Ecuador" "Egypt"
#> [39] "El Salvador" "Equatorial Guinea"
#> [41] "Eritrea" "Estonia"
#> [43] "Federal Republic of Somalia" "Fiji"
#> [45] "Finland" "France"
#> [47] "Gabon" "Gambia"
#> [49] "Georgia" "Germany"
#> [51] "Ghana" "Greece"
#> [53] "Grenada" "Guatemala"
#> [55] "Guinea" "Guinea-Bissau"
#> [57] "Guyana" "Haiti"
#> [59] "Honduras" "Iceland"
#> [61] "India" "Indonesia"
#> [63] "Iran" "Iraq"
#> [65] "Ireland" "Israel"
#> [67] "Italy" "Ivory Coast"
#> [69] "Jamaica" "Japan"
#> [71] "Jordan" "Kazakhstan"
#> [73] "Kenya" "Kiribati"
#> [75] "Kuwait" "Latvia"
#> [77] "Lebanon" "Liberia"
#> [79] "Libya" "Lithuania"
#> [81] "Madagascar" "Malaysia"
#> [83] "Maldives" "Malta"
#> [85] "Marshall Islands" "Mauritania"
#> [87] "Mexico" "Micronesia"
#> [89] "Monaco" "Montenegro"
#> [91] "Morocco" "Mozambique"
#> [93] "Myanmar" "Namibia"
#> [95] "Nauru" "Netherlands"
#> [97] "New Zealand" "Nicaragua"
#> [99] "Nigeria" "North Korea"
#> [101] "Norway" "Oman"
#> [103] "Pakistan" "Palau"
#> [105] "Palestine" "Panama"
#> [107] "Papua New Guinea" "Peru"
#> [109] "Philippines" "Poland"
#> [111] "Portugal" "Qatar"
#> [113] "Republic of Mauritius" "Republic of the Congo"
#> [115] "Romania" "Russia"
#> [117] "Saint Kitts and Nevis" "Saint Lucia"
#> [119] "Saint Vincent and the Grenadines" "Samoa"
#> [121] "Sao Tome and Principe" "Saudi Arabia"
#> [123] "Senegal" "Seychelles"
#> [125] "Sierra Leone" "Singapore"
#> [127] "Slovenia" "Solomon Islands"
#> [129] "South Africa" "South Korea"
#> [131] "Spain" "Sri Lanka"
#> [133] "Sudan" "Suriname"
#> [135] "Sweden" "Syria"
#> [137] "São Tomé and Principe" "Taiwan"
#> [139] "Tanzania" "Thailand"
#> [141] "Togo" "Tonga"
#> [143] "Trinidad and Tobago" "Tunisia"
#> [145] "Turkey" "Turkmenistan"
#> [147] "Tuvalu" "Ukraine"
#> [149] "United Arab Emirates" "United Kingdom"
#> [151] "United States" "Uruguay"
#> [153] "Vanuatu" "Venezuela"
#> [155] "Vietnam" "Western Sahara"
#> [157] "Yemen"
#>
#> $mrgid_ter2
#> [1] 2103 2121 2123 2124 2126 2129 2133 2147 2149 2166 2175 2177
#> [13] 2180 2185 2186 2188 2190 2194 2204 2215 2240 2253 3297 5343
#> [25] 5363 5660 8597 8606 8607 8613 8617 8623 8624 8638 8640 8652
#> [37] 8685 9011 14864 20312 36288 48934 48935 48936 48994 48995 48996 49593
#> [49] 64457 64458
#>
#> $mrgid_sov2
#> [1] 15 17 2103 2121 2123 2124 2126 2129 2131 2133 2147 2149 2157 2163 2166
#> [16] 2175 2180 2185 2186 2188 2190 2194 2202 2204 2208 2215 2221 2226 2240 2252
#> [31] 2253 3868 7963 8600 8603 8640 8685
#>
#> $territory2
#> [1] "Abu musa, Greater and Lesser Tunb"
#> [2] "Alhucemas Islands"
#> [3] "Argentina"
#> [4] "Aruba"
#> [5] "Australia"
#> [6] "Cayman Islands"
#> [7] "Ceuta"
#> [8] "Chafarinas Islands"
#> [9] "Colombia"
#> [10] "Dominican Republic"
#> [11] "Doumeira Islands"
#> [12] "Ecuador"
#> [13] "Faeroe"
#> [14] "Falkland / Malvinas Islands"
#> [15] "Galapagos"
#> [16] "Glorioso Islands"
#> [17] "Guinea-Bissau"
#> [18] "Guyana"
#> [19] "Halaib Triangle"
#> [20] "Honduras"
#> [21] "Iceland"
#> [22] "Ile Tromelin"
#> [23] "Iran"
#> [24] "Israel"
#> [25] "Italy"
#> [26] "Jan Mayen"
#> [27] "Japan"
#> [28] "Kuril Islands"
#> [29] "Liancourt Rocks"
#> [30] "Libya"
#> [31] "Matthew and Hunter Islands"
#> [32] "Mayotte"
#> [33] "Melilla"
#> [34] "Navassa Island"
#> [35] "Nigeria"
#> [36] "Perejil Island"
#> [37] "Peñón de Vélez de la Gomera"
#> [38] "Russia"
#> [39] "Saudi Arabia"
#> [40] "Senkaku Islands"
#> [41] "Singapore"
#> [42] "Slovenia"
#> [43] "South Georgia and the South Sandwich Islands"
#> [44] "Spain"
#> [45] "Sweden"
#> [46] "Taiwan"
#> [47] "Trinidad and Tobago"
#> [48] "Ukraine"
#> [49] "United States"
#> [50] "Wake Island / Enenkio"
#> [51] "Western Sahara"
#>
#> $iso_ter2
#> [1] "ABW" "ARG" "ATF" "AUS" "COL" "CYM" "DOM" "ECU" "ESH" "ESP" "FLK" "FRO"
#> [13] "GNB" "GUY" "HND" "IRN" "ISL" "ISR" "ITA" "JPN" "LBY" "MYT" "NGA" "RUS"
#> [25] "SAU" "SGP" "SGS" "SJM" "SVN" "SWE" "TTO" "TWN" "UKR" "UMI" "USA"
#>
#> $sovereign2
#> [1] "Argentina" "Australia" "China"
#> [4] "Colombia" "Comores" "Denmark"
#> [7] "Djibouti" "Dominican Republic" "Ecuador"
#> [10] "Egypt" "France" "Guinea-Bissau"
#> [13] "Guyana" "Honduras" "Iceland"
#> [16] "Iran" "Israel" "Italy"
#> [19] "Japan" "Libya" "Madagascar"
#> [22] "Marshall Islands" "Morocco" "Netherlands"
#> [25] "Nigeria" "Norway" "Russia"
#> [28] "Saudi Arabia" "Singapore" "Slovenia"
#> [31] "South Korea" "Spain" "Sweden"
#> [34] "Trinidad and Tobago" "United Kingdom" "United States"
#> [37] "Vanuatu"
#>
#> $mrgid_ter3
#> [1] 2201 2206 8613 8640 20312 48936
#>
#> $mrgid_sov3
#> [1] 2201 2206 8603 8614 8640 8682
#>
#> $territory3
#> [1] "Dominican Republic" "Ile Tromelin" "Senkaku Islands"
#> [4] "United Arab Emirates" "Venezuela"
#>
#> $iso_ter3
#> [1] "ARE" "DOM" "VEN"
#>
#> $sovereign3
#> [1] "China" "Dominican Republic" "Republic of Mauritius"
#> [4] "United Arab Emirates" "Venezuela"
#>
#> $x_1
#> [1] "-0.85027" "-1.96130" "-10.17763" "-10.92284" "-10.98204"
#> [6] "-105.33346" "-107.43869" "-109.21728" "-11.20987" "-11.75544"
#> [11] "-119.97660" "-127.42131" "-139.85200" "-14.14468" "-14.36337"
#> [16] "-144.00327" "-15.62051" "-151.05753" "-154.72634" "-16.81091"
#> [21] "-160.46839" "-161.30341" "-162.89881" "-169.06347" "-169.33138"
#> [26] "-169.50612" "-17.12724" "-17.56720" "-17.61396" "-17.69542"
#> [31] "-17.91755" "-172.08495" "-172.45397" "-172.75372" "-174.76586"
#> [36] "-176.72727" "-176.81123" "-18.38751" "-18.44845" "-18.50357"
#> [41] "-18.77443" "-2.19330" "-2.45356" "-2.49561" "-2.91697"
#> [46] "-24.17164" "-27.97601" "-29.09644" "-3.87866" "-31.26381"
#> [51] "-31.97096" "-39.44423" "-4.15801" "-4.28845" "-4.30609"
#> [56] "-4.57203" "-5.21961" "-5.25129" "-5.30551" "-5.33333"
#> [61] "-5.42011" "-5.45221" "-5.70869" "-51.75618" "-53.32143"
#> [66] "-54.76698" "-55.16096" "-56.38701" "-57.13214" "-57.64094"
#> [71] "-57.88712" "-57.99356" "-58.32237" "-59.85044" "-59.98478"
#> [76] "-6.99690" "-60.20055" "-60.57064" "-60.72114" "-61.10359"
#> [81] "-61.69442" "-62.05881" "-62.15567" "-62.48384" "-62.54329"
#> [86] "-62.62740" "-62.90578" "-63.12285" "-63.12987" "-63.20282"
#> [91] "-63.60185" "-64.50497" "-64.80693" "-64.92435" "-65.91423"
#> [96] "-66.78687" "-66.84125" "-68.36978" "-68.97176" "-69.65326"
#> [101] "-69.67224" "-69.67341" "-7.51858" "-70.85361" "-71.09015"
#> [106] "-72.50386" "-73.54856" "-75.18373" "-75.57785" "-75.62881"
#> [111] "-77.29089" "-78.06222" "-78.63333" "-78.88478" "-79.14572"
#> [116] "-79.85562" "-79.99086" "-8.50000" "-80.30185" "-80.34583"
#> [121] "-81.13914" "-81.16088" "-81.43712" "-81.53050" "-82.20864"
#> [126] "-82.53293" "-83.49562" "-83.84845" "-83.94222" "-86.50737"
#> [131] "-87.56601" "-88.21289" "-88.84132" "-89.74411" "-89.94236"
#> [136] "-9.85201" "-90.83174" "-92.09080" "-95.82133" "1.82135"
#> [141] "10.50095" "10.59577" "10.73256" "102.54228" "103.82677"
#> [146] "104.45239" "105.36523" "108.75025" "109.27469" "11.03537"
#> [151] "11.05319" "113.38608" "114.10176" "117.89121" "118.14570"
#> [156] "118.71458" "12.28547" "12.93234" "122.16379" "124.12211"
#> [161] "124.27075" "126.85410" "127.57712" "127.57808" "128.99535"
#> [166] "13.30933" "13.57896" "131.86633" "133.06950" "138.08411"
#> [171] "138.44863" "14.00130" "142.40882" "144.00261" "145.73933"
#> [176] "147.96154" "15.11121" "15.64779" "150.32248" "150.71973"
#> [181] "158.65291" "163.20377" "163.68539" "166.12396" "166.53046"
#> [186] "167.48547" "167.92898" "168.55743" "17.26042" "17.59256"
#> [191] "172.03655" "172.78214" "18.11402" "18.30277" "18.35677"
#> [196] "18.68015" "19.11300" "2.42123" "2.72132" "20.36307"
#> [201] "21.35288" "21.88919" "23.00232" "23.93215" "24.44331"
#> [206] "25.70163" "29.20165" "3.53979" "30.07850" "31.15948"
#> [211] "32.63526" "33.22060" "33.63465" "34.10220" "34.23444"
#> [216] "34.94186" "34.94683" "35.49624" "36.87917" "37.46457"
#> [221] "37.80854" "37.95740" "38.14367" "39.42518" "4.18649"
#> [226] "4.82275" "40.10928" "40.48313" "40.56174" "40.59255"
#> [231] "41.16860" "41.93765" "42.09428" "43.22221" "43.43296"
#> [236] "43.45423" "45.30656" "47.09690" "47.25395" "48.49205"
#> [241] "48.58199" "48.61916" "48.69255" "5.43745" "5.58699"
#> [246] "50.33172" "50.70534" "51.01440" "51.21209" "51.59986"
#> [251] "51.88076" "52.06161" "52.46872" "52.63853" "53.87936"
#> [256] "53.89303" "54.67305" "54.71328" "55.17180" "58.66998"
#> [261] "6.35335" "60.11720" "64.71175" "68.97460" "7.58768"
#> [266] "71.72934" "71.99388" "73.08545" "73.73051" "74.28785"
#> [271] "76.08526" "77.54181" "8.39609" "8.73897" "81.63607"
#> [276] "9.04886" "9.20529" "9.31089" "90.56153" "92.18967"
#> [281] "93.47078" "93.67735" "94.65552" "96.85434" "99.62852"
#>
#> $y_1
#> [1] "-0.04184" "-0.25912" "-0.59113" "-0.91400" "-0.96556" "-1.37183"
#> [7] "-10.04465" "-11.09799" "-11.24358" "-11.42314" "-11.61810" "-11.70110"
#> [13] "-11.89940" "-12.03146" "-12.75492" "-13.21698" "-13.29687" "-13.85484"
#> [19] "-15.01891" "-15.71127" "-15.97278" "-16.58523" "-16.81021" "-17.30663"
#> [25] "-17.94847" "-18.73634" "-19.52315" "-20.22175" "-20.37062" "-20.50277"
#> [31] "-20.86054" "-20.91335" "-21.01871" "-21.78197" "-23.27911" "-23.53682"
#> [37] "-23.64969" "-24.60623" "-26.33091" "-26.82400" "-27.36226" "-29.09314"
#> [43] "-3.06254" "-3.30578" "-3.38855" "-3.47464" "-3.73181" "-3.82154"
#> [49] "-33.50802" "-35.78927" "-36.25493" "-38.29431" "-38.76190" "-40.98629"
#> [55] "-42.76615" "-46.30782" "-46.83292" "-47.05868" "-48.87795" "-5.33648"
#> [61] "-5.45663" "-51.66703" "-53.49863" "-54.87630" "-56.52404" "-6.40153"
#> [67] "-6.54778" "-6.55593" "-7.21087" "-7.81254" "-7.94747" "-8.63257"
#> [73] "-9.04989" "-9.53965" "-9.78156" "0.77910" "0.79130" "1.27144"
#> [79] "1.40778" "1.46058" "10.15136" "10.22543" "10.30869" "10.56539"
#> [85] "10.58066" "10.68079" "10.95788" "11.26100" "11.60379" "11.84859"
#> [91] "11.99710" "12.24388" "12.26791" "12.55243" "12.71120" "12.75889"
#> [97] "12.78887" "12.81975" "12.88906" "12.92958" "13.14179" "13.15805"
#> [103] "13.16246" "13.32288" "13.32867" "13.69463" "13.74138" "13.87770"
#> [109] "14.30362" "14.38087" "14.59807" "14.97512" "14.99651" "15.01851"
#> [115] "15.05334" "15.30381" "15.47035" "15.49739" "15.79719" "15.82346"
#> [121] "15.84999" "16.09190" "16.12316" "16.41292" "16.66861" "16.75109"
#> [127] "16.79880" "16.91154" "16.99022" "17.23444" "17.25496" "17.33890"
#> [133] "17.37739" "17.79821" "17.91219" "17.93270" "17.93813" "18.03469"
#> [139] "18.24852" "18.28660" "18.33814" "18.70111" "18.73279" "18.94915"
#> [145] "19.13826" "19.15345" "19.50326" "19.74792" "2.06760" "2.46352"
#> [151] "20.01803" "20.38988" "20.47561" "20.89448" "21.35018" "21.42076"
#> [157] "21.60004" "22.45276" "22.61164" "22.80987" "23.34236" "23.53950"
#> [163] "24.04099" "24.69243" "24.94396" "25.11716" "25.86236" "26.01269"
#> [169] "26.33540" "26.52080" "27.73853" "28.36715" "29.17480" "29.43517"
#> [175] "29.55656" "29.75582" "29.78160" "29.87235" "3.22641" "3.30891"
#> [181] "3.46278" "3.50201" "3.80280" "30.44760" "30.81396" "31.56915"
#> [187] "32.36899" "32.61120" "32.78135" "32.81282" "33.15017" "33.17302"
#> [193] "33.95296" "34.48929" "34.76211" "35.16990" "35.18525" "35.23422"
#> [199] "35.31355" "35.31672" "35.32871" "35.74071" "35.86909" "35.91465"
#> [205] "36.00133" "36.08832" "36.50720" "37.20344" "37.24233" "38.31921"
#> [211] "38.47538" "39.29232" "39.65266" "39.82785" "4.08512" "4.63949"
#> [217] "4.84155" "40.28350" "40.52224" "40.92708" "41.30715" "41.72201"
#> [223] "41.87023" "42.44625" "42.91656" "42.93396" "43.34802" "43.43699"
#> [229] "43.88606" "44.24109" "44.47442" "44.95192" "45.25417" "45.49770"
#> [235] "45.56287" "45.85719" "46.07075" "49.12645" "49.58296" "5.02226"
#> [241] "5.16367" "51.46321" "52.65304" "53.61335" "54.18818" "54.55382"
#> [247] "54.91230" "55.75616" "56.15007" "56.79247" "57.10508" "58.77012"
#> [253] "58.80998" "59.01170" "59.48460" "6.06282" "6.44393" "6.54638"
#> [259] "6.63541" "6.68090" "6.68794" "6.73691" "6.76843" "60.03609"
#> [265] "61.18112" "61.75484" "62.59460" "63.15298" "64.69826" "68.17846"
#> [271] "68.58756" "69.29167" "7.59776" "71.11421" "71.32185" "72.03395"
#> [277] "72.92452" "75.47965" "78.71360" "8.28880" "8.60754" "8.61338"
#> [283] "9.16729" "9.18114" "9.97738"
#>
#> $mrgid_eez
#> [1] 3293 5668 5669 5670 5672 5673 5674 5675 5676 5677 5678 5679
#> [13] 5680 5681 5682 5683 5684 5685 5686 5687 5688 5689 5690 5691
#> [25] 5692 5693 5694 5695 5696 5697 8308 8309 8310 8311 8312 8313
#> [37] 8314 8315 8316 8317 8318 8319 8321 8322 8323 8324 8325 8326
#> [49] 8327 8328 8331 8332 8333 8334 8337 8338 8339 8340 8341 8343
#> [61] 8345 8346 8347 8348 8349 8350 8351 8352 8353 8354 8355 8356
#> [73] 8357 8358 8359 8360 8361 8362 8363 8364 8365 8366 8367 8368
#> [85] 8369 8370 8371 8372 8373 8374 8375 8376 8378 8379 8380 8381
#> [97] 8382 8383 8384 8385 8386 8387 8388 8389 8390 8391 8392 8393
#> [109] 8394 8395 8396 8397 8398 8400 8401 8402 8403 8404 8405 8406
#> [121] 8407 8408 8409 8411 8412 8413 8414 8415 8416 8417 8418 8419
#> [133] 8420 8421 8423 8424 8425 8426 8427 8428 8429 8430 8431 8432
#> [145] 8433 8435 8437 8438 8439 8440 8441 8442 8443 8444 8445 8446
#> [157] 8447 8448 8449 8450 8451 8452 8453 8454 8455 8456 8457 8459
#> [169] 8460 8461 8462 8463 8464 8465 8466 8467 8468 8469 8470 8471
#> [181] 8472 8473 8474 8475 8476 8477 8478 8479 8480 8481 8482 8483
#> [193] 8484 8485 8486 8487 8488 8490 8491 8492 8493 8494 8495 8758
#> [205] 21787 21788 21789 21790 21791 21792 21796 21797 21798 21803 22491 22756
#> [217] 26517 26518 26519 26520 26521 26522 26523 26524 26526 26582 33177 33178
#> [229] 33179 33180 33181 33185 48943 48944 48945 48946 48947 48948 48950 48951
#> [241] 48952 48953 48954 48955 48956 48957 48961 48962 48964 48965 48966 48967
#> [253] 48968 48969 48970 48971 48972 48973 48974 48975 48976 48977 48978 48980
#> [265] 48982 48984 48985 48997 48998 48999 49000 49001 49002 49003 50167 50170
#> [277] 62589 62596 62598 64430 64431 64440 64446 64459 64460
#>
#> $iso_sov1
#> [1] "AGO" "ALB" "ARE" "ARG" "ATG" "AUS" "AZE" "BEL" "BEN" "BGD" "BGR" "BHR"
#> [13] "BHS" "BIH" "BLZ" "BRA" "BRB" "BRN" "CAN" "CHL" "CHN" "CIV" "CMR" "COD"
#> [25] "COG" "COL" "COM" "CPV" "CRI" "CUB" "CYP" "DEU" "DJI" "DMA" "DNK" "DOM"
#> [37] "DZA" "ECU" "EGY" "ERI" "ESH" "ESP" "EST" "FIN" "FJI" "FRA" "FSM" "GAB"
#> [49] "GBR" "GEO" "GHA" "GIB" "GIN" "GMB" "GNB" "GNQ" "GRC" "GRD" "GTM" "GUY"
#> [61] "HND" "HRV" "HTI" "IDN" "IND" "IRL" "IRN" "IRQ" "ISL" "ISR" "ITA" "JAM"
#> [73] "JOR" "JPN" "KAZ" "KEN" "KHM" "KIR" "KNA" "KOR" "KWT" "LBN" "LBR" "LBY"
#> [85] "LCA" "LKA" "LTU" "LVA" "MAR" "MCO" "MDG" "MDV" "MEX" "MHL" "MLT" "MMR"
#> [97] "MNE" "MOZ" "MRT" "MUS" "MYS" "NAM" "NGA" "NIC" "NLD" "NOR" "NRU" "NZL"
#> [109] "OMN" "PAK" "PAN" "PER" "PHL" "PLW" "PNG" "POL" "PRK" "PRT" "PSE" "QAT"
#> [121] "ROU" "RUS" "SAU" "SDN" "SEN" "SGP" "SLB" "SLE" "SLV" "SOM" "STP" "SUR"
#> [133] "SVN" "SWE" "SYC" "SYR" "TGO" "THA" "TKM" "TLS" "TON" "TTO" "TUN" "TUR"
#> [145] "TUV" "TWN" "TZA" "UKR" "URY" "USA" "VCT" "VEN" "VNM" "VUT" "WSM" "YEM"
#> [157] "ZAF"
#>
#> $iso_sov2
#> [1] "ARG" "AUS" "CHN" "COL" "COM" "DJI" "DNK" "DOM" "ECU" "EGY" "ESP" "FRA"
#> [13] "GBR" "GNB" "GUY" "HND" "IRN" "ISL" "ISR" "ITA" "JPN" "KOR" "LBY" "MAR"
#> [25] "MDG" "MHL" "NGA" "NLD" "NOR" "RUS" "SAU" "SGP" "SVN" "SWE" "TTO" "USA"
#> [37] "VUT"
#>
#> $iso_sov3
#> [1] "ARE" "CHN" "DOM" "MUS" "VEN"
#>
#> $un_sov1
#> [1] 8 12 24 28 31 32 36 44 48 50 52 56 70 76 84 90 96 100
#> [19] 104 116 120 124 132 144 152 156 158 170 174 178 180 188 191 192 196 204
#> [37] 208 212 214 218 222 226 232 233 242 246 250 262 266 268 270 275 276 288
#> [55] 296 300 308 320 324 328 332 340 352 356 360 364 368 372 376 380 384 388
#> [73] 392 398 400 404 408 410 414 422 428 430 434 440 450 458 462 470 478 480
#> [91] 484 492 499 504 508 512 516 520 528 548 554 558 566 578 583 584 585 586
#> [109] 591 598 604 608 616 620 624 626 634 642 643 659 662 670 678 682 686 690
#> [127] 694 702 704 705 706 710 724 729 732 740 752 760 764 768 776 780 784 788
#> [145] 792 795 798 804 818 826 834 840 858 862 882 887
#>
#> $un_sov2
#> [1] 32 36 158 170 174 208 214 218 250 262 328 340 352 364 376 380 392 410 434
#> [20] 450 504 528 548 566 578 584 624 643 682 702 705 724 752 780 818 826 840
#>
#> $un_sov3
#> [1] 156 214 388 480 784 862
#>
#> $un_ter1
#> [1] 8 12 16 24 28 31 32 36 44 48 50 52 56 60 70 76 84 90
#> [19] 92 96 100 104 116 120 124 132 136 144 152 156 158 162 166 170 174 175
#> [37] 178 180 184 188 191 192 196 204 208 212 214 218 222 226 232 233 234 238
#> [55] 239 242 246 250 254 258 260 262 266 268 270 275 276 288 292 296 300 304
#> [73] 308 312 316 320 324 328 332 334 340 352 356 360 364 368 372 376 380 384
#> [91] 388 392 398 400 404 408 410 414 422 428 430 434 440 450 458 462 470 474
#> [109] 478 480 484 492 499 500 504 508 512 516 520 528 531 533 534 535 540 548
#> [127] 554 558 566 570 574 578 580 581 583 584 585 586 591 598 604 608 612 616
#> [145] 620 624 626 630 634 638 642 643 652 654 659 660 662 663 666 670 678 682
#> [163] 686 690 694 702 704 705 706 710 724 732 740 744 752 760 764 768 772 776
#> [181] 780 784 788 792 795 796 798 804 818 826 831 832 833 834 840 850 858 862
#> [199] 876 882 887
#>
#> $un_ter2
#> [1] 32 36 136 156 170 175 214 218 234 238 239 260 328 352 364 376 380 392 533
#> [20] 566 624 643 682 705 724 732 744 752 780 804 840
#>
#> $un_ter3
#> [1] 214 784 862
#>
# Get EEZ area just for Bermuda
get_area(area_name = "Bermuda", mregions_column = "territory1")
#> Simple feature collection with 1 feature and 31 fields
#> Geometry type: POLYGON
#> Dimension: XY
#> Bounding box: xmin: -68.91706 ymin: 28.90577 xmax: -60.7048 ymax: 35.80855
#> Geodetic CRS: WGS 84
#> # A tibble: 1 × 32
#> mrgid geoname mrgid_ter1 pol_type mrgid_sov1 territory1 iso_ter1 sovereign1
#> <int> <chr> <int> <chr> <int> <chr> <chr> <chr>
#> 1 8402 British E… 8636 200NM 2208 Bermuda BMU United Ki…
#> # ℹ 24 more variables: mrgid_ter2 <int>, mrgid_sov2 <int>, territory2 <chr>,
#> # iso_ter2 <chr>, sovereign2 <chr>, mrgid_ter3 <int>, mrgid_sov3 <int>,
#> # territory3 <chr>, iso_ter3 <chr>, sovereign3 <chr>, x_1 <dbl>, y_1 <dbl>,
#> # mrgid_eez <int>, area_km2 <int>, iso_sov1 <chr>, iso_sov2 <chr>,
#> # iso_sov3 <chr>, un_sov1 <dbl>, un_sov2 <dbl>, un_sov3 <dbl>, un_ter1 <dbl>,
#> # un_ter2 <dbl>, un_ter3 <dbl>, geometry <POLYGON [°]>
# Get EEZ areas for Kiribati (iso_ter1 = KIR)
get_area(area_name = "KIR", mregions_column = "iso_ter1")
#> Simple feature collection with 3 features and 31 fields
#> Geometry type: MULTIPOLYGON
#> Dimension: XY
#> Bounding box: xmin: -180 ymin: -13.83833 xmax: 180 ymax: 7.879056
#> Geodetic CRS: WGS 84
#> # A tibble: 3 × 32
#> mrgid geoname mrgid_ter1 pol_type mrgid_sov1 territory1 iso_ter1 sovereign1
#> <int> <chr> <int> <chr> <int> <chr> <chr> <chr>
#> 1 8450 Kiribati … 8658 200NM 2116 Phoenix G… KIR Kiribati
#> 2 8441 Kiribati … 8657 200NM 2116 Line Group KIR Kiribati
#> 3 8488 Kiribati … 17597 200NM 2116 Gilbert I… KIR Kiribati
#> # ℹ 24 more variables: mrgid_ter2 <int>, mrgid_sov2 <int>, territory2 <chr>,
#> # iso_ter2 <chr>, sovereign2 <chr>, mrgid_ter3 <int>, mrgid_sov3 <int>,
#> # territory3 <chr>, iso_ter3 <chr>, sovereign3 <chr>, x_1 <dbl>, y_1 <dbl>,
#> # mrgid_eez <int>, area_km2 <int>, iso_sov1 <chr>, iso_sov2 <chr>,
#> # iso_sov3 <chr>, un_sov1 <dbl>, un_sov2 <dbl>, un_sov3 <dbl>, un_ter1 <dbl>,
#> # un_ter2 <dbl>, un_ter3 <dbl>, geometry <MULTIPOLYGON [°]>